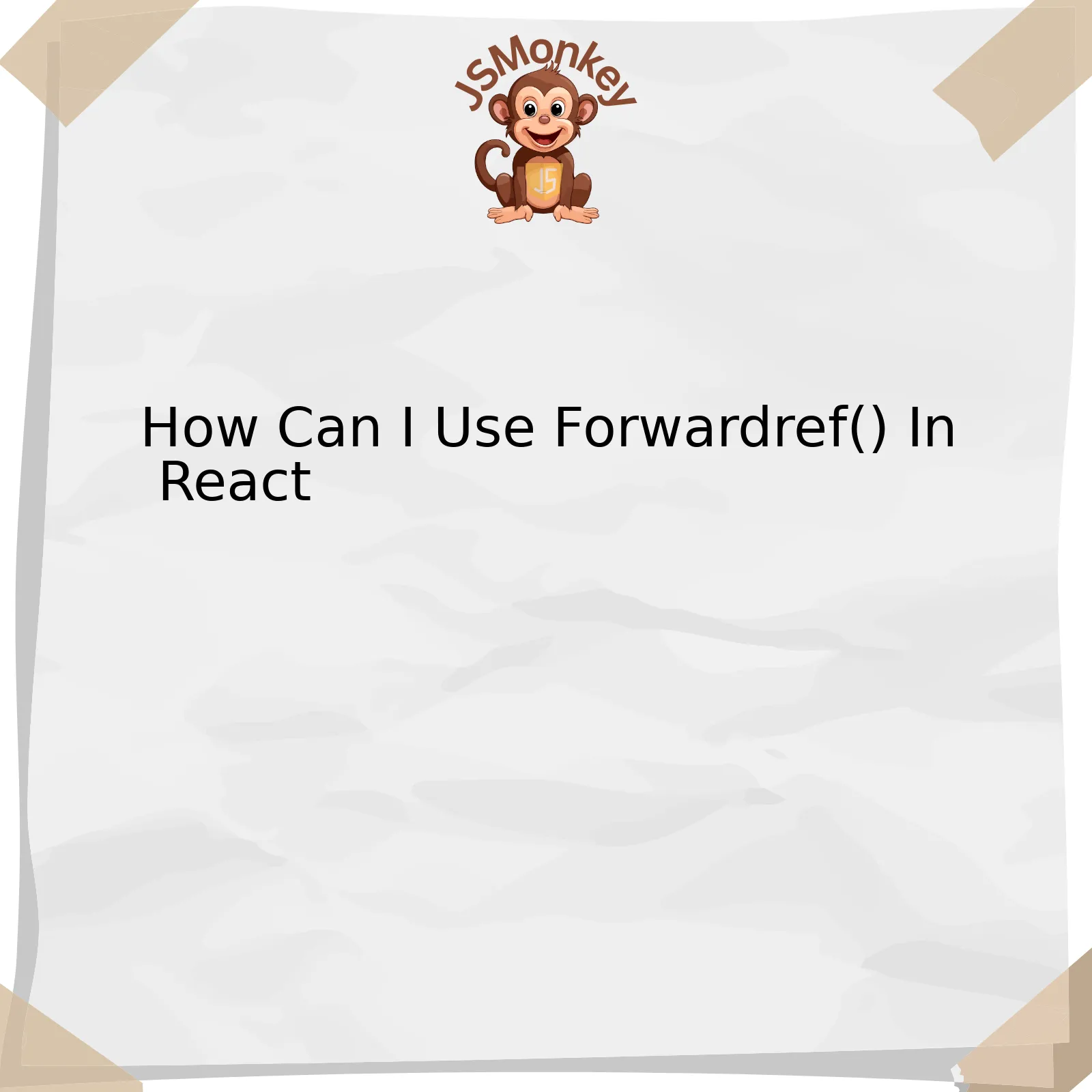
ForwardRef in React offers an advanced feature that allows you to access a child component’s properties directly from a parent component. Utilizing the `React.forwardRef()` function, you can provide a ‘ref’ (reference) to a child component and manipulate it as per your requirement in a parent component.
The use of ForwardRef is especially beneficial in cases where you require more substantial control over child elements or when certain events occur in your application. In addition, this functionality provides an excellent way for developers to manage form inputs, media playback controls, or animations within their application effectively.
Here is how ForwardRef is singularly beneficial in numerous ways:
Situation | Benefit |
---|---|
Flexible components | The ForwardRef allows a parent component to interact with its children, allowing flexible component creation and enhanced customization. |
Managing form inputs | You may want to focus on a text input field automatically; using the forwardRef lets you call DOM methods without needing to add state logic to the child component. |
Media Playback Controls | If you’re working with video rendering or audio features, you may need specific playback controls. Using forwardRef, you can directly control a child component from a parent one. |
To demonstrate, let’s assume we have a child component `ChildComponent` that we’d like to be able to “focus” on from the parent component `ParentComponent`. We can accomplish this by utilizing the React.forwardRef() function.
In `ChildComponent`:
const ChildComponent = React.forwardRef((props, ref) => ( <input type="text" ref={ref} /> ));
Then, in `ParentComponent`:
function ParentComponent() { const childRef = React.useRef(); function onClick() { // Here we can directly access the `focus()` method because it's forwarded. childRef.current.focus(); } return ( <div> <ChildComponent ref={childRef} /> <button onClick={onClick}>Focus Input</button> </div> ); }
This way, the parent component has direct control over the child input element.
To embrace social impact in technology, in the wise words of Steve Jobs. “Technology is nothing. What’s important is that you have faith in people, they’re basically good and smart, and if you give them tools, they’ll do wonderful things with them.This quote resonates appropriately with ForwardRef functionality in React. It serves as an essential tool for developers to create robust user interfaces.
Understanding the Purpose and Functionality of ForwardRef() in React
The
forwardRef()
function in React is a highly beneficial tool that allows a component to transfer its received ‘ref’ from its props directly to a child component. This results in the parent component having direct access to the raw DOM node or the instance of the child component.
Now, walking hand-in-hand with the initial question about understanding the purpose and functionality of
forwardRef()
, let’s segue into how one can effectively utilize
forwardRef()
in their React application.
Step 1: Import ForwardRef
You’ll first need to import
forwardRef
from ‘react’. See the code snippet below:
import React, { forwardRef } from 'react';
Step 2: Creating a Child Component using ForwardRef
Create a child component that uses the
forwardRef()
function to assign the passed ref to an HTML element. Here’s an example of how you can accomplish this:
const ChildComponent = forwardRef((props, ref) => ( ));
Step 3: Parents Assigning Refs
Next, inside the parent component, create a reference using the
React.createRef()
method and then pass it to the ChildComponent like shown below:
class ParentComponent extends React.Component { inputRef = React.createRef(); render() { return; } }
With these steps, any parent components of ChildComponent are now able to directly interact with the
input
element. This interaction could be something as straightforward as setting the focus on the input field.
Further reading regarding this topic can be found in the official React documentation, which offers more comprehensive knowledge about possible usage scenarios and potential caveats with
forwardRef()
.
A notable quote by Douglas Crockford comes to mind when dealing with intricate concepts such as these, “Programming is the most complicated thing that humans know how to do.”
Exploring Real-World Examples of ForwardRef() Usage in React
The
forwardRef()
function in React, more commonly known as Forward Ref, deals with directing references (‘refs’) to their child components. Often needed when access to a specific attribute or method from a child component is required, that might otherwise be unavailable due to the encapsulation provided by the parent-child component interaction in the default React architecture.
A Real-world example of ForwardRef usage:
Consider the case where we have a child component called InputComponent that includes an input field. Now, we have a parent component called FormComponent that manages Form Component behavior and holds a submit button. On clicking the submit button, you want to focus back on the input field that is present within the InputComponent. This would involve directly manipulating the DOM, and this is where
forwardRef()
becomes useful.
This hypothetical case can be addressed using
forwardRef()
in the following manner:
Define your child component, InputComponent, using
forwardRef()
:
html
;
))} />
Here, we are forwarding a ref from ParentComponent to InputComponent to get direct access to the text input’s DOM element present inside InputComponent.
And then we create a ref in FormComponent using React.createRef() method, which we later pass as a prop to InputComponent when it gets declared:
html
On clicking ‘Submit’, the reference created by
React.createRef()
, inputEl, allows us to directly call the focus method on the DOM element, resulting in the desired behavior of focusing back on the input field inside InputComponent.
As Jordan Walke, one of the principal software engineers at Facebook and a co-creator of React once said – “There is a lot of value in breaking from convention if it means pursuing something meaningful in its place”. This quote applies well here as moving away from conventional parent-child interactions in React and using
React.forwardRef()
, offers more control over the child components, adding meaningful and desired functionality to our applications.
Remember that, while
forwardRef()
empowers manipulation of child components directly, its usage should be sparing, mainly when common parent-child interaction won’t suffice. Overuse can result in hard-to-follow code and compromise the modularity benefits that React otherwise provides. Lastly, all references should be managed responsibly to avoid memory leaks. Visit this link for more details.
In-depth Breakdown: Implementing ForwardRef() into a React Application
The `forwardRef()` function is a valuable and practical feature provided by the React library. By assisting you in passing a `ref` through a component to one of its child components, it significantly improves your react application’s interactivity and user experience. This function caters to scenarios where you need to modify a child component directly and are unable to do so due to the encapsulation provided by higher-order components.
To comprehend how to use this function, let’s explore its functionality in detail:
Element | Description |
---|---|
forwardRef() Function |
This is a method provided by React that allows `refs` to be forwarded to the child element. |
Props in React | These are parameters supplied to a React component either from a parent or within the component itself. |
`ref` Attribute | A special attribute provided by React that can be attached to any component. It helps to interact with the component and access its properties directly. |
Let’s observe a classic example of using `forwardRef()`:
Assume that we have a parent component called `ParentComponent`, and a child input element that we would like to focus on when our application starts.
Passing a simple ref to the child won’t suffice. Instead, we make use of the `forwardRef()` function in the `ChildComponent`.
html
const ChildComponent = React.forwardRef((props, ref) => (
));
class ParentComponent extends React.Component {
constructor(props){
super(props);
this.childRef = React.createRef();
}
componentDidMount() {
this.childRef.current.focus();
}
render() {
return
}
}
In the above example, `forwardRef()` receives a function as parameter where `props` remain the usual properties passed to the component and `ref` holds the reference value. This reference can then be connected to any desired element.
As Kent C. Dodds says, “Code is like humor. When you have to explain it, it’s bad.” Learning about react’s `forwardRef()` function might seem a bit confusing at first glance, the trick lies in practicing and continuously working with it. Start incorporating this feature into your React applications and before long, you will master its utility and implementation.
Potential Challenges and Solutions with ForwardRef() in React
When working with
forwardRef()
in React, there are several potential challenges to be aware of. However, these challenges can be overcome by adopting the correct implementation strategies.
Firstly, understanding how
forwardRef()
works can initially pose a challenge given its unique approach to passing references.
Potential Challenge:
The foremost challenge is understanding that React’s
forwardRef()
function is not similar to regular component properties. It doesn’t return a component, but it provides a way to pass refs down through a component to one of its child components or DOM elements.
Solution:
To overcome this, developers should understand that
forwardRef()
creates a React component that forwards the
ref
attribute received from a parent component to its child component. This technique becomes really helpful when you’re creating reusable component libraries.
Here is a typical scenario where you might want to use
forwardRef()
:
html
const ButtonElement = React.forwardRef((props, ref) => (
));
This `ButtonElement` component uses `forwardRef()` to take a `ref` passed to it and attach it to the actual DOM button element.
Strong>Have a look on official documentation for Forward Ref.
Secondly, using
forwardRef()
increases complexity, which may result in debugging difficulties.
Potential Challenge:
Little or no warnings are presented when issues arise in components utilizing
forwardRef()
, making them harder to debug. Furthermore, as refs interact directly with DOM elements; hence, incorrect use can manipulate the DOM in unexpected ways.
Solution:
To mitigate this challenge, React introduced
React.forwardRef()
, which erases much of the complexity. Remember to only use `
forwardRef()` when necessary, such as needing direct access to DOM elements or managing focus, text selection, or media playback.forwardRef()is special, and thus it might take a little while to wrap your head around it. But mastering it can considerably improve your React skills. As Donald Knuth, a renowned computer scientist said, "Science is knowledge which we understand so well that we can teach it to a computer." This is wholly applicable to the use of
forwardRef()in React - the more you understand it, the more efficiently you will use it.
Overall, understanding and properly implementing
forwardRef()in React takes practice and experience. Revisiting the documentation on refs and the
forwardRef()function will keep enhancing your comprehension and usage of it.
Making use of the 'forwardRef()' functionality within React allows developers to access the properties and capabilities of a child component from the parent component, thereby expanding the realm of possibilities in complex web application development.Forwardref enhances element reference handling in react by forwarding refs to the DOM or class component. In other words,
React.forwardRef()acts as a conduit, providing deep-seated access to underlying elements that might otherwise be inaccessible. It is particularly beneficial when working with user interfaces demanding precise control over focus behavior, text selection, or media playback.
The basic syntax for using forwardRef in React would generally take the following form:
const FancyButton = React.forwardRef((props, ref) => ( ));
In this scenario, `FancyButton` is a component which forwards its ref to the native button DOM element. This allows `FancyButton`’s parents to directly interact with the underlying button’s DOM node. Hence, React `forwardRef()` adds another layer of potential for React developers looking to build powerful, interactive components.
Indeed, as renowned software engineer Linus Torvalds noted, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” (source). The utilization of techniques like `forwardRef()` effectively encapsulates this sentiment, offering intrigue and broadened horizons to the world of JavaScript and React developers.