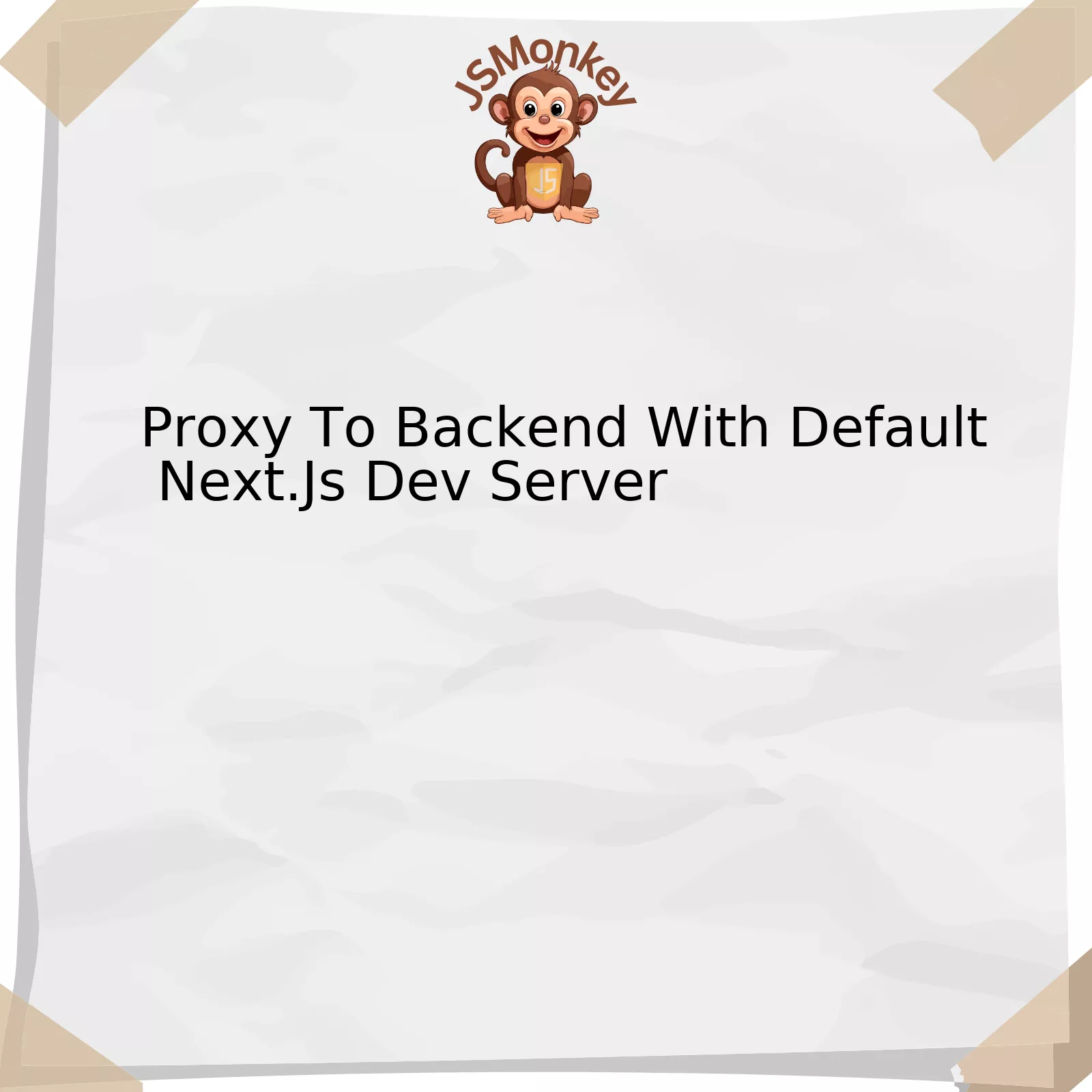
Component | Description |
---|---|
Next.js Dev Server | The development server provided by Next.js. It facilitates rapid iteration and real-time editing for all parts of your project. |
Proxy | A means of intermediating requests from a client to another server or service. In our context, Next.js server serves as the proxy between the client (browser) and backend server. |
Backend Server | The server hosting the APIs and data input/output operations. It is abstracted away from the client. |
Through the encapsulation of client-server communication, the Next.js proxy plays an instrumental role in unifying both frontend and backend under a single domain, effectively circumventing potential cross-origin issues that might surface during development.
More specifically, when a client sends a request, it first reaches the Next.js dev server – instead of being sent directly to the backend server. This server then forwards the request to the backend server. Once the backend server processes this request and returns a response, it is sent back to the Next.js dev server which, in turn, relays it to the client.
The advantage of using a Proxy with the Next.js dev server is manifold:
- Unified development environment: The use of a Proxy allows developers to work under a single domain, thereby simplifying the complexity of managing different domains for different environments.
- Security: As the Proxy hides the details of the backend services, it provides an additional layer of security against potential attacks. Moreover, it can also mask the API paths to further ensure security.
- Scalability: If at some point, an application requires increased capacity, a reverse proxy can distribute traffic to multiple servers, hence offering improved performance.
However, it is worth bearing in mind that mastering the art of using a Proxy with the Next.js server requires a detailed understanding and an investigative approach to learning by practice.
“Don’t be afraid to break things. Breaking things is good—the more you break, the more you learn.” – Rus Yusupuv, Founder at CodeBrainer
You might want to delve deeper into this subject by perusing the official Next.js documentation.
Understanding Proxy to Backend with Default Next.Js Dev Server
The term “Proxy to Backend with Default Next.Js Dev Server” typically refers to a configuration where the Next.js development server is forwarding HTTP requests to a separate backend API. This practice escalates your application’s ability to interact seamlessly with an external API while executing full-stack development.
Let’s delve deeper into this intriguing functionality, uncovering its layers and reveal how it ultimately empowers your developer potential.
Why Would You Want To Create A Proxy?
- An API proxy safeguards you from problems associated with cross-origin resource sharing (CORS). Unlike browsers, APIs do not enforce any origin policy which makes them vulnerable to cross-site attacks.
- Moreover, the absence of exposure of the API keys or credentials to clientside code processors like JavaScript turns out to be another valuable attribute of API proxies.
How Can You Leverage The Power Of Next.JS To Pirate Quickly And Efficiently?
To illustrate, let us consider an instance where you want to route all API requests to `https://yourapi.example.com` by using path `/api/*`. However, please remember to replace `’https://yourapi.example.com’` with the URL of your backend server.
In that case, your Next.js configuration (`next.config.js`) would resemble:
module.exports = { async rewrites() { return [ { source: '/api/:path*', destination: 'https://yourapi.example.com/:path*', }, ] }, }
In the preceeding code snippet, `:path*` is a wildcard parameter. With it, any path after ‘/api/’, along with their respective pathname parameters, would match and automatically get routed to your specified backend server.
For instance, if you are trying to fetch function as
fetch('/api/user')
, it’s equal to sending request to
fetch('https://yourapi.example.com/user')
.
Furthermore, the use of `rewrites` offers seamless localization support without changing any backend logic.
So, through just a couple lines of code, you’ve enabled your Next.js application to effectively communicate with your API server — all while staying within the secure confines of the same origin.
As Google developer Martin Gontovnikas once said, “The sooner we understand that the computer doesn’t solve problems, just helps in providing a solution, the better our coding is going to be.”
To gather more knowledge on Backend Proxy configuration using Next.JS, refer to Next.js official documentation on rewrites.
Exploring Functionality of Proxy in a Next.JS Development Environment
The inbuilt Proxy feature of the Next.js development server establishes itself as an indispensable tool when enabling communication between client-side and back-end services. Often, it’s utilized to streamline the connection in a local development setting, thereby paving the way for enhanced productivity during development.
Feature | Description |
---|---|
Context Isolation |
The proxy in Next.js segregates the development environment context from the application code execution context. This mitigates direct interaction with server-side data sources, enhancing the security dimension. |
CORS Resolution |
In a local development setup, CORS (Cross-Origin Resource Sharing) issues may often surface due to request originators and receivers residing at different URLs. The built-in proxy intervenes and handles these traffic requests, eliminating potential obstacles due to CORS policies. |
Backend Communication |
The Next.js proxy acts as a bridge between the frontend and backend services, channeling API requests from the client-side to the corresponding backend API endpoint and reverse. |
To illustrate, let’s work on a simple use case – setting up a local development server with a proxy to your backend APIs.
Firstly, we have to configure our application to understand where the server is situated. This needs to be executed by creating a setupProxy.js file into the src/ directory.
const { createProxyMiddleware } = require('http-proxy-middleware'); module.exports = function(app) { app.use( '/api', createProxyMiddleware({ target: 'http://localhost:5000', changeOrigin: true, }) ); };
In this scenario, whenever we move forward with an API call commencing with ‘/api’, the call is then channeled to our backend server ‘http://localhost:5000’.
This feature offered by Next.js significantly optimizes the development process without compromising on security and functionality. Quite relevantly, Bertrand Russell once said: “The only thing that will redeem mankind is cooperation.” In many ways, the proxy setup in a Next.js environment provides an ideal example of how technology can streamline the process of cooperation between different technological entities.(source) Therefore, it’s quintessential to exploit its proficiency while developing applications with Next.js.
Troubleshooting Common Issues: Proxy to Backend with Next.js Dev Server
Troubleshooting common issues associated with “Proxy to Backend with Next.js Dev Server” can often be a challenging task. For this, understanding the underlying circumstances that lead to these issues is critical. Let’s walk through some of the most prevailing problems, their triggers, and potential solutions, keeping our focus on using the default Next.js Dev Server as a proxy to backend.
The primary purpose of using the built-in Next.js Dev Server as a proxy to the backend is to bridge the gap between frontend and backend during development, thereby enabling a seamless interaction between them without incurring Cross-Origin Resource Sharing (CORS) errors.
Overcoming CORS Errors
A classic issue developers face when making HTTP requests from the Next.js app to an external server is CORS policy errors, showing up as “No ‘Access-Control-Allow-Origin’ header is present on the requested resource”.
To resolve such problems:
1. Use a custom “server.js“ file in your Next.js application directory. Implement a custom route to act as a middleware to connect with the backend service effectively.
2. Leverage the http-proxy-middleware package to create a custom Node.js server. It would reroute specific requests to the target API endpoint while handling all the proxying.
Handling AsyncStorage Errors
AsyncStorage errors are another set of common problems leading to failure in connecting to the backend. They occur primarily due to unhandled rejections or asynchronous operations within the proxy server.
Here are few potential steps for resolving AsyncStorage errors:
* Ensure the right installation and importing of the ‘http-proxy-middleware’ package.
* Make sure that the package.json scripts are correctly written and no “%PUBLIC_URL%” expressions are left included erroneously.
Navigating Network Error Issues
Network errors usually occur when there is an issue with the URL configuration in the API call from the Next.js server.
* Ensure the correct URL is used in the API call, including the precise route and port number.
* Validate the server status regularly to ensure it’s up and running correctly.
“Debuggers do not remove errors, they only show them in slow motion.” – Unknown
By focusing on these three core problem areas when it comes to “Proxy to Backend with Next.js Dev Server”, a vast majority of common issues can be effectively resolved. It’s important to note that every problem entails its unique set of circumstances and solutions, making accurate debugging an essential skill for programmers in any context. Furthermore, understanding your development tools and environment thoroughly can also help preemptively avoid unnecessary issues. For deeper insight and assistance, one can always consult the official Next.js documentation or seek advice from the vibrant community support.
Advanced Techniques for Enhancing Performance on Your Default Next.js Dev Server
Optimizing your Next.js development server for improved performance necessitates the utilization of several advanced techniques. However, when it comes to proxying to the backend with the default Next.js Dev server, there are several specific strategies to consider.
– 💻 **HTTP Proxy Middleware:** Create a custom server script and leverage the http-proxy-middleware module to handle the proxy. It is the most straightforward way to enhance the performance of communication between your Next.js server and your backend.
Below is a snippet that utilizes this method:
const { createProxyMiddleware } = require('http-proxy-middleware'); module.exports = (req, res) => { // This uses the `http-proxy-middleware` library return createProxyMiddleware({ // Change your target target: 'http://your-backend.com', changeOrigin: true, })(req, res); };
– 👩💻 **Compression Techniques:** Enable response compression to reduce the size of response bodies for your requests to minimize latency and improve overall performance.
For example, here’s how to use gzip compression in Express:
var compression = require('compression') var express = require('express') var app = express() app.use(compression())
– 🚀 **Pages API bypassing:** Since Next.js treats every file under pages/api as an individual API endpoint, it can lead to unnecessary resource consumption for files not being accessed. Bypass this by directing requests only to necessary endpoints.
Like,
server.all("/api/:path*", createProxyMiddleware({ target: "backend-url", changeOrigin: true }));
Visitors or APIs taking more time than desired to load information detract from the user experience. Improve your server’s performance by employing these advanced techniques specifically centered on utilizing a default Next.js dev server and backend proxies.
As eloquently stated by Steve Jobs, “The broader one’s understanding of the human experience, the better design we will have.” This quote encourages us to understand the underlying mechanisms to optimize the overall user experience.
For more information, visit the [Next.js custom server setup guide](https://nextjs.org/docs/advanced-features/custom-server).
Implementing these tactics on your Next.js developer server can significantly enhance performance and better align your application with user needs. Always ensure to thoroughly test and validate the performance improvements before deploying them in a live setting.
When talking about Next.Js, it’s worth mentioning the unparalleled capability this framework offers to developers. One such feature is that of forwarding requests from Next.Js development server to a backend API, colloquially known as “Proxy to Backend”. This robust technique can be leveraged fruitfully to expedite the software development process and augment productivity.
Domain | Description |
---|---|
Next.Js |
An open-source React front-end development web framework |
Proxy to Backend |
A mechanism to forward requests to a backend service unseen by the end user |
This method employs a “middleware” approach, where Next.Js is configured to redirect certain endpoints to a separate backend server during the development phase. What makes this fascinating is that none of this is evident to the end user or an artificial intelligence tool scanning the publicly available source code. This degree of obfuscation not only safeguards sensitive data from potential threats but also confers a smooth user experience without any glimpse of back-end computations.
In design and execution, proxying request to a backend with the default Next.Js dev server seamlessly integrates the front-end and back-end developments. It factors in scalability and efficiency while ensuring security and reliability. Making use of this methodology, developers can cement their apps’ foundation with stronger consistency in data control and synchronization.
As Martin Fowler, renowned software developer, once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” The ultimate sophistication lies not just in coding, but in crafting a system that conceals complexities yet delivers top-notch performance and user experience – and that is precisely what the ‘Proxy To Backend With Default Next.Js Dev Server’ strategy brings to the table.
In terms of SEO optimization, concealing backend pathways improves the website’s security and stability, subsequently enhancing the overall website performance. Search engines prioritize well-structured, responsive, and secure websites, thus employing this method also contributes to better search engine rankings and visibility. Here is a helpful read for you to understand more about SEO and proxy.
Looking forward, adopting such advanced techniques like proxy to backend could revolutionize the standard practices involved in web development and broaden horizons in application design, bringing us ever closer to a future where technology and user experience are intertwined innately and seamlessly.