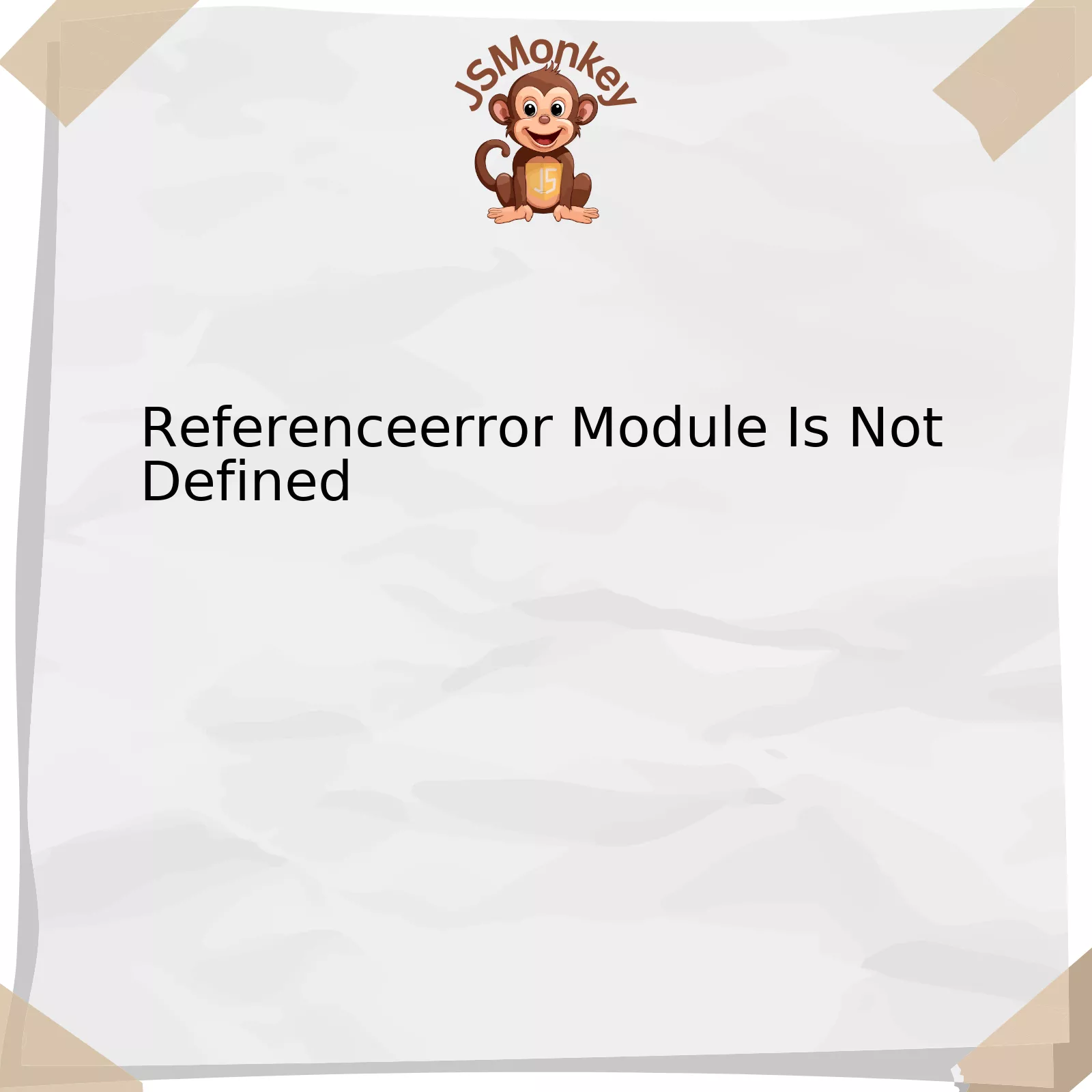
The “ReferenceError: Module is not defined” error in Javascript results from attempted access to a module which either does not exist or has not been loaded or defined within the context of its use. It’s one of conventional errors that JavaScript developers encounter frequently.
As the name suggests, ReferenceErrors concern attempts to refer to some variable quantity or method which is unknown or not currently available within execution context.
Error Type | Example | Possible Cause | Suggested Solution |
---|---|---|---|
Undefined Module in Node.js |
const myModule = require('myModule'); |
‘myModule’ either doesn’t exist or is not located within the accessible scope of your project. | Use npm to install ‘myModule’ if it’s missing, or check your file paths if it exists but isn’t being found. |
Not Defined Global Scope |
console.log(myGlobalVar); |
‘myGlobalVar’ was not defined in the global scope. | Declare ‘myGlobalVar’ in the global scope or import it where necessary. |
Not Imported Correctly |
import { myFunc } from 'myModule'; |
Either ‘myModule’ doesn’t export a method named ‘myFunction’, or perhaps ‘myModule’ wasn’t imported correctly. | Check ‘myModule’ to ensure it exports ‘myFunction’, or review the import statement for possible errors. |
To avoid such error, it is crucial to do a diligent check on the following:
- The spelling of your modules. Simple typo in the module name can result in Javascript failing to recognize them.
- Correct usage of imports and exports. Ensure that the module is properly exported at the source and accurately imported where it’s needed.
- Installation of packages. For external modules, ensure you have installed them using npm or Yarn and they exist in your node_modules folder.
It’s worth noting that understanding the core reason behind this common error would go a long way in preventing similar issues in the future. As Steve McConnell once said, “Good code is its own best documentation.”
Understanding the “ReferenceError Module is Not Defined” Error
ReferenceError: Module is Not Defined
is a common issue encountered by JavaScript developers. This error typically signifies that you’re making an attempt to utilize a variable or function which hasn’t been defined in the current scope.
However, let’s delve deep into understanding the
ReferenceError: Module is Not Defined
error and the process to solve it.
## Origin of the Error
JavaScript
ReferenceErrors
occur as a result of using undefined identifiers at runtime. When your code tries to call a non-existent module, JavaScript will throw a ReferenceError due to its inability to find this particular module. These can be methods, functions, or variables that are assumed to exist in your codebase but haven’t been created yet, or they might be out of scope.
For example,
html
Here, we tried to log
myModule
onto the console. However, because
myModule
has not yet been declared, JavaScript throws up a
ReferenceError: myModule is Not Defined
.
## Resolving ReferenceError: Module is Not Defined
When encountering a
ReferenceError: Module is Not Defined
, there are various strategies you may use to resolve it:
– **Check your spelling**: It may sound trivial, but a quick double-check on the exact spelling of your module is always prudent.
– **Module loading order**: Make sure that dependent modules are loaded in the correct order. Some modules have dependencies on others, therefore they should be loaded after their dependencies.
– **Using
typeof
Operator**: Prior to invoking or assigning a function/module, check if it exists using the typeof operator.
For instance:
html
In the example above, we first check if
myModule
actually exists before attempting to log it onto the console.
As Brian Kernighan once said _”Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”_ So remember, when debugging these issues taking a systematic approach and breaking things down step by step will inevitably guide you to solution.
Exploring Causes of the “Module Not Defined” Error in Coding
Examining the origins of the “Module Not Defined” error in coding can be instrumental in resolving issues related to this incessantly reoccurring error. This error is typically denoted as “Referenceerror Module Is Not Defined” and often bewilders programmers due to its potential arising from diverse causes.
The top two causes tied with the “Referenceerror Module Is Not Defined” error include:
- Incorrect Exporting of Modules
- Failed Import of Modules
Modules in JavaScript are standalone pieces of code that are logically separated but can be accessed and used by other modules. A module should be appropriately exported using
module.exports
or
exports
, enabling it to be accessible from other files.
When a particular module isn’t correctly exported, you may encounter the “module not defined” error.
Example:
// incorrect function helloWorld() { console.log("Hello World!"); } // correct module.exports = { helloWorld: function() { console.log("Hello World!") } }
Problems might occur while importing the defined and exported modules into another file. You must use the
require()
function to ensure proper import of a module. Failing to do so will trigger the same “module not defined” error.
Example:
// incorrect const hello = 'helloWorld'; // correct const hello = require('./helloWorld');
A great quote I came across from Steve Jobs elucidates on the debugging process related to such errors: “You can’t just ask customers what they want and then try to give that to them. By the time you get it built, they’ll want something new.” (source)
In other words, it’s often up to us as developers to stay ahead of the game, anticipate issues (like “Referenceerror Module Is Not Defined”) before they happen and adeptly manage them when they do. Troubleshooting these errors aids us in achieving a more profound understanding of JavaScript’s working mechanism, subsequently leading to robust and efficient code.
When the root causes leading to such erring behaviors are determined, as revealed in this discourse, the troubleshooting process becomes markedly simplified and less time-consuming. Hence, getting familiar with different common error types is an integral part of honing your coding skills.
Resolving the Issue: Strategies to Deal with Undefined Modules
The issue
Referenceerror Module Is Not Defined
typically arises when you attempt to reference a module that has not been defined. This can be the result of several factors, including:
– Incorrect file paths
– Misspelling or mistaken capitalization in the module name
– Errors in your code that mistakenly call an undefined variable or object.
Resolving this issue often depends on the specific context and circumstances that cause the error. Here are some strategies to consider:
1. Check for Correct File Paths
Ensure that you’re correctly targeting the location of the module. If your file paths aren’t accurate, JavaScript won’t be able to find the module, resulting in the “Module is not defined” ReferenceError. An example of how file paths might be defined can be seen below:
<script src="./moduleFolder/myModule.js"></script>
In certain instances, it might be necessary to explicitly define your directory routes depending on how your project is organized.
2. Verify Spelling and Capitalization
JavaScript is case sensitive, so mistakes in spelling or capitalization can be culprits of the issue. Always verify if the module’s name referred in your code matches exactly with the actual file’s name.
3. Examine Your Code
Another possible scenario could be a missing
exports
statement in the module’s file that makes it available to other files in your project. Adding an exports statement like below can resolve the issue:
module.exports = MyModule;
Additionally, inspect your code for potential logical errors where an undefined object or variable has been erroneously called, triggering the error.
It’s important to remember that MDN’s JavaScript Error Reference is a beneficial resource to comprehend various error messages, helping debug encountered issues.
As Douglas Crockford, the creator of JSON and a respected figure in the world of JavaScript once said: “Programming is the most complicated thing that humans do. Debugging must be equally complicated.”. Indeed, knowing how to effectively debug an issue such as
Referenceerror Module Is Not Defined
, takes patience and practice, but it results in better understanding and mastery over programming.
Preventive Measures: Avoiding Future ‘Module Not Defined’ Errors
Experiencing a ‘Module Not Defined’ error in JavaScript can be quite frustrating. This error message typically indicates that there’s an issue regarding the scope of the module, which might not be correctly defined or may not even exist at all. In order to avoid this issue from occurring later, some preventive measures can be adopted.
1. Ensuring Appropriate Scope and Definition:
Before you make use of any module for your project, ensure it has been declared appropriately. A common practice is using Node.js’s
require()
method or ECMAScript 6 (ES6)’s
import
feature to import modules. For instance, if you’re attempting to employ a module named ‘express’, your code must include the line
var express = require('express');
or
import * as express from 'express';
if you’re using ES6 syntax.
2. Detecting Errors Early:
Endeavor to run unit tests frequently throughout your coding process. Regular tests can assist in catching errors, such as “Module Not Defined”, in a way that allows rectification earlier rather than later when it becomes apportionment of larger problems. Tools like Jest or Mocha offer an accessible environment for testing JavaScript codes.
3. Being Wary of Third-Party Modules:
Use third-party modules with caution. They might not be defined or could have bugs that prevent them from being imported correctly. Always check for module compatibility with your current JavaScript environment.
4. Proper Named Imports:
When employing ES6 syntax, the named imports must correspond exactly to the named exports within the module. Make sure the names are correct since these are case sensitive.
5. Understanding Asynchronous Module Definitions (AMDs):
Some projects use AMDs, which stands for Asynchronous Module Definition. Understanding the concept of AMD is crucial if you wish to avoid unforeseen errors related with modules.
These steps are all vital preventive measures that can avert the ‘Module Not Defined’ error from occurring in your applications. While corrective actions can solve current issues, adopting a more preventive approach contributes significantly towards reducing future occurrences.
As Donald Knuth, a renowned computer scientist once said, “Let us change our traditional attitude to the construction of programs: Instead of imagining that our main task is to instruct a computer what to do, let us concentrate rather on explaining to human beings what we want a computer to do”. The act of defining modules correctly and systematically coincides with this vision, as it simplifies the process not just for the computers executing the code, but also for other developers who might need to understand, edit or debug the code later on.
For a deeper dive into module systems and their definition, you may wish to peruse this MDN Web Docs guide.
The phrase
ReferenceError: Module is not defined
is a common roadblock that can hinder the development process in Javascript programming. Such an error appears when trying to access or utilize a module that has not been previously declared, loaded or defined within your script.
• Understanding the Context: A module is essentially a discrete chunk of code that is stored in its own separate file and may contain variables, functions, classes, or even other modules. Modules are critical for maintaining clean, organized code, particularly in large-scale applications.
• Interpreting the Error: The error message ‘Module is Not Defined’ typically surfaces in one of the following scenarios:
– Attempting to call a function from a module without importing it.
– Incorrect syntax or typos in the module’s filename or import statement.
– Erroneously presuming that certain modules are part of JavaScript’s core language, when they actually need to be installed via NPM or another package manager.
The crucial aspect to resolve this issue would be to first locate where exactly this module was initially expected to be defined. From there, determining whether it was supposed to be imported from another file or whether it was unintentionally left undefined will be important.
Visualizing the error message:
Error Message | Possible Cause | Solution |
---|---|---|
ReferenceError: Module is not defined |
Attempting to use a module that has not been imported | Import the necessary module into the script |
ReferenceError: Module is not defined |
Typographical error in module filename or import statement | Verify the exact spelling and syntax of all filenames and import/export statements |
ReferenceError: Module is not defined |
Incorrect assumption that certain modules are available natively in JavaScript | Install the necessary modules via NPM or another package manager |
At first glance, tackling a ‘Module is Not Defined’ error might seem intimidating. However, with the above understanding and knowing what to look for, the task of debugging becomes much more manageable.
As Douglas Crockford, a renowned JavaScript developer and author, once said, “Programming is the act of installing internal, pre-established reactions into the computers.” Encountering errors like this one is just a part of that very process – shaping and correcting our program as per it’s required behavior.