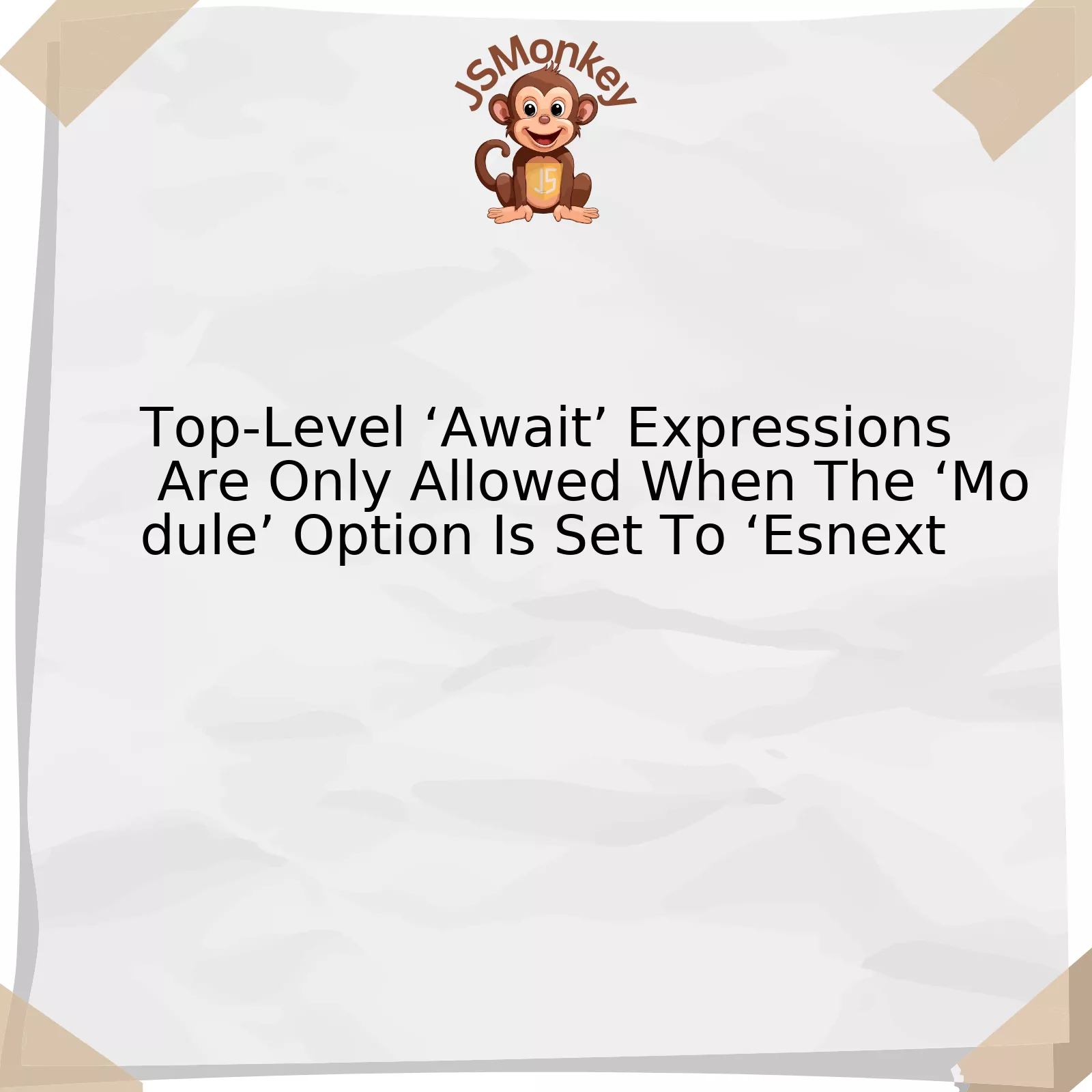
The concept of ‘Await’ expressions at the top-level and its relation with the ‘Module’ option when set to ‘Esnext’ can be understood as follows:
Concept | Description |
---|---|
Top-Level ‘Await’ Expressions | The phenomenon where JavaScript’s ‘Await’ keyword is used outside of asynchronous functions directly within modules. The primary idea behind this technique is to give developers more flexibility in handling asynchronous operations. |
‘Module’ Option Set To ‘Esnext’ | An option setting in TypeScript configuration which instructs the transpiler to treat the file to be compiled as a module that has the most recently added specifications, in other words – the next generation JavaScript features. Setting this to ‘Esnext’ enables top level await syntax. |
The significance of this concept emerges from its utility in simplifying asynchronous code. JavaScript developers traditionally use async functions or Promises to handle asynchronous code execution. However, both methods often lead to the “Pyramid of Doom” complexity issue when dealing with multiple asynchronous processes sequentially.
By allowing ‘Await’ expressions at the top-level, developers can write cleaner, less nested code. It makes it easier to write and read asynchronous code, benefiting particularly in instances of script loading, dynamic imports, or fetching resources.
To put it into context, consider the following example:
Without top-level await:
(async () => { const response = await fetch('API URL'); const data = await response.json(); // Use `data` })();
With top-level await:
const response = await fetch('API URL'); const data = await response.json(); // Use `data`
As evident, the latter reduces nesting and increases readability.
However, top-level ‘Await’ expressions are only allowed when the ‘Module’ option is set to ‘Esnext’. It essentially indicates that your development environment acknowledges ECMAScript module syntax with the latest features, such as dynamic import or optional chaining.
Google’s developer Jake Archibald resonates this concept stating, “Top-level await works best where the module doing the awaiting doesn’t provide exports used by other modules.”
To summarise, while it contributes towards better asynchronous code management, its usage should be carefully considered based on the project requirement to avoid unnecessary performance costs.
Exploring the Functionality of Top-Level ‘Await’ Expressions
The top-level `await` expressions are an essential part of Javascript, particularly in module-based systems. By definition, a “top-level await” is an `await` keyword outside of any function, class, or other structures that can occur at the base level of the module. This improvement in ECMAScript syntax enables us to use async components and procedures at the root level, simplifying the process for developers handling promises in their code.
Notably, there’s an important criterion to meet when using this innovative functionality: Top-level `await` expressions are only permissible if the ‘module’ option is configured to ‘esnext’ in your project settings. To understand why, we need to interpret its rationale:
– The top-level `await` operator was conceived primarily to operate under modules. Modules run independently allowing the developer to have better control and understanding of the execution time of each module.
– The ‘esnext’ signifies that state-of-the-art syntax rules are being employed. The ‘esnext’ option includes support for dynamic imports, top-level `await`, and other modern features that emerge in Javascript. By setting the ‘module’ option to ‘esnext’, the project is effectively configured to handle futuristic functionalities like top-level `await`.
In the JavaScript ecosystem, setting up your environment to use these options might look something as displayed below:
{ "compilerOptions": { "target": "esnext", "module": "esnext", } }
Remember, it means using the `await` keyword at the very topmost scope of a module. Here’s a practical example:
import fetchSomething from 'anyModule' const data = await fetchSomething(); console.log(data);
Stepping forward with fresh advancements in technology while enabling greater usability, the myriad possibilities of top-level `await` are being explored. As Brendan Eich, co-founder of Mozilla, and Creator of JavaScript puts it, “Always bet on JavaScript.” It emphasizes the potential of JavaScripts to continually evolve, utilizing its dynamic capabilities that cater to developer’s needs.source. The continuous enrichment of Javascript’s syntax, such as this one with top-level `await`, is an embodiment of this quote.
Decoding ‘Module’ Options: Understanding Its Connection with ‘ESNext’
Understanding the link between ‘Module’ options and ‘ESNext’, along with their relationship to top-level ‘await’ expressions can be a bit perplexing. However, let’s break it down into manageable parts.
First, we need to understand what a ‘module’ is. In JavaScript, the term ‘module’ refers to a distinct piece of code that can be utilized multiple times within a larger program without polluting the global namespace (source). Essentially, by utilizing modules, you can avoid variable name clashes and collisions in your projects.
On the other hand, ‘ESNext’ is a term used to denote features of JavaScript that have been proposed for future revisions of the ECMAScript standard. ESNext includes syntax and features that are not officially part of a finalized ES6 or later version yet, but will likely be included in future iterations of the language.
In terms of the top-level ‘await’ expressions, they refer to effectively pausing execution at the top level of the module, until the promise-based asynchronous operation has completed. There is a specific reason for them being exclusively allowed when the ‘Module’ option is set to ‘ESNext’. To encapsulate this, we need to dive into how these three concepts interrelate.
Until recently, using ‘await’ on the top-level was a syntax error. It could only be used inside an ‘async function’. This meant that developers had to wrap top-level promises in an immediately-invoked async function (
(async () => {...})()
). However, with the introduction of top-level ‘await’, there’s no longer a need to wrap asynchronous operations in an async function.
The twist here is, this feature (top-level ‘await’) is exclusively allowed when the ‘Module’ option is set to ‘ESNext’. In other words, the compiler expects ECMAScript module syntax and semantics (the top-level await being part of them) when you mention ‘module: esnext’ in your tsconfig.json.
And here’s why:
- It allows developers to use the latest, proposed features of JavaScript which includes the top-level await.
- The new ES modules (esnext) in JavaScript has provisions for syntax like import/export statements and they also support top-level await, hence the connection.
Here’s an example of how this can be executed:
//asynchronously fetch data const data = await fetch('https://api.example.com/data');
This kind of direct ‘await’ expression at the top-level of the code is facilitated by setting the ‘Module’ option to ‘ESNext’. This setting fits well with the ethos of JavaScript development, which constantly evolves to accommodate easier, more intuitive coding practices.
As Ada Lovelace, a pioneer in computer programming affirmed, “The Analytical Engine has no pretensions to originate anything. It can do whatever we know how to order it to perform”. The advancements like ‘ESNext’ and top-level ‘await’ offer programmers the ability to instruct machines effectively and expressively.
Implications of Using ‘Await’ in JavaScript’s ESnext Module
The `await` operator in JavaScript serves a crucial role as it helps to pause and resume the JavaScript execution while waiting for a promise. Initially, `await` was only allowed inside `async` functions. However, with ESnext features, top-level `await` can be used directly at the top level of modules, significantly alleviating difficulties developers might have faced previously.
Top-Level ‘Await’ Expressions
Just as it states, top-level `await`\ expressions refers to the use of the await keyword outside of async functions, essentially at the top level of your code. It impacts both the module’s evaluation phase and its network loading phase due to the necessary pause for awaiting resolution of promises.
For example, the following form of coding without the `module` option set to `Esnext` would be deemed incorrect:
let value = await fetchData(); console.log(value);
Setting the `module` option to `ESNext` now makes this type of coding feasible.
Implications and Relevance to Your Code Structure
1. Fewer Unnecessary Wrappers – Prior to this feature, `await` being used outside an `async` function required wrapping it in an immediately invoked function expression (IIFE), which could bring about unwanted indirect changes to the scope and lead to more complex, harder-to-read/write code.
2. Fetching Resources – Loading dependencies from network requests, assets, or I/O often takes time, so `await` comes in handy by ensuring the necessary resources are available before executing any dependent code, thus reducing code errors.
3. Module Evaluation Serialization – The top-level ‘await’ operates linearly rather than concurrently on each module that utilizes this element. This provides a significant performance implication, especially when dealing with many modules simultaneously.
4. Enhanced Error Handling – While trying to `await` a module that fails to load, JavaScript doesn’t proceed to evaluate the rest of the code. This leads to a better understanding of potential issues, fewer runtime crashes, and easier debugging.
Notwithstanding, top-level `async`\ functions have their perils as well, thus, thoughtful planning should be given whenever they are employed in programming practice.
Talking about programming, Bill Gates once said, “Everyone should learn how to program a computer because it teaches you how to think.”source As we embrace ESNext’s features, remember that strong programming skills always matter, as they help you write cleaner, more efficient code. ‘Await’ only goes further to make this truth evident.
Detailing the Restrictions and Flexibility Offered by Top-Level ‘Await’ and ‘ESNext’.
Let us delve into the coding universe and explore top-level `await` and `ESNext`. Both are pivotal concepts for JavaScript developers. These two features offer certain restrictions and a great deal of flexibility, strengthening and enhancing the user experience.
The `await` keyword, a more recent introduction to the JavaScript landscape, is primarily used in an Async/await function. However, it can only be used at the top level when the `module` option is set to ‘ESNext’. This condition serves as our springboard into discussing how these elements come together to form an intricate web of possibilities in the world of coding.
First, we need to decode the term `module` here. The module system in JavaScript assists in breaking down large and complex codes into multiple manageable modules or files. Each module has a specific job, enhancing readability and reusability in bigger projects. Thus, setting the `module` option as ‘ESNext’ forms an important step in facilitating JavaScript language’s evolution by supporting new syntax, types, APIs, objects, statements, or expressions like the top-level `await`.
In ESNext, a top-level `await` allows you not just to use `await` in an `async function`, but also lets you conveniently use it at the top level of modules. This ability can drastically simplify work with modules that return promises. The caveat, however, is that while it lends itself to code’s clarity and efficiency, this option could possibly limit parallelism, especially when multiple scripts are importing a single module.
Consider the following idea:
// This will throw an error await Promise.resolve(console.log('Hello, World!')); // But this won't, when the `module` option is set to 'ESNext' import message from './message_module'; console.log(message);
In the example, the first block will raise an error because `await` cannot exist at the top level outside of ESNext. The second block, on the contrary, will not lead to error and we’ll experience a seamless run when we set the `module` option as ‘ESNext’.
While it can look like top-level `await` might stall performance as it forces code to run synchronously, it also lessens the reliance on constructing self-executing functions or creating unnecessary wrappers around your code just for awaiting promises. This balance grants JavaScript developers much-desired flexibility while ensuring they operate within some restrictions for better optimization.
In Bill Gates words: “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” (Brainy quote). And that’s what `await` and `ESNext` strive to achieve: smoothing intricate processes and enhancing the overall coding experience.
Expanding upon the topic of using top-level ‘await’ expressions, one might discover that these are only permissible when the ‘module’ option is set to ‘esnext’. This stands as a cardinal rule in Javascript programming. The introduction of asynchronous functions and the ‘await’ operator has truly revolutionized Javascript, bringing some significant changes to how we work with this well-established language.
In accordance with ECMAScript specification, `await` can solely be used within an `async` function. However, top-level `await` allows its usage at the top level of modules. This feature betters performance by reducing the need for intermediate IIFEs or asynchronous workarounds. In effect, it permits developers to unequivocally write clean and efficient code.
Setting the ‘module’ option to ‘esnext’ activates several features including top-level ‘await’. For instance:
// This will give an error await Promise.resolve(console.log('Hello')); // Uncaught SyntaxError: await is only valid in async function
To resolve this error, set the ‘module’ option to ‘esnext’. Remember that this might require altering the compiler configuration settings in your IDE or adjusting your project’s build tool configuration.
Not but not least, take heed of the words of Martin Fowler, a renowned programmer and co-author of the Agile Manifesto, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Remember that in this constantly evolving technological world, understanding and adapting to the nuances of these function calls and configurations like setting ‘module’ options to ‘esnext’ to allow top-level ‘await’ expressions, is what will help elevate us from being just good programmers to exceptional ones.
For more understanding on this concept, the Mozilla Developer Network’s detailed coverage on ‘await’ is a stellar resource.