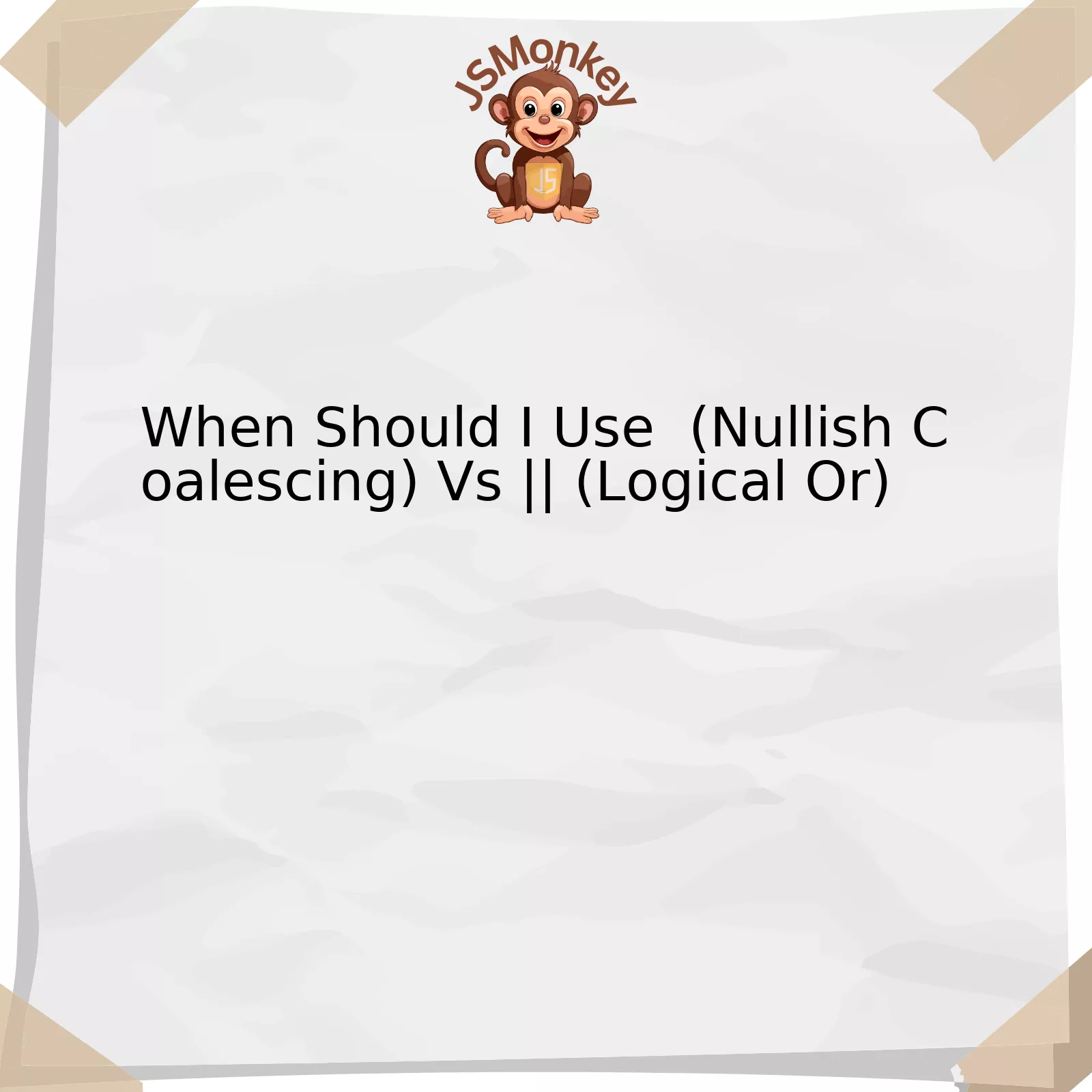
Operator | When to Use |
---|---|
Nullish Coalescing Operator (??) | Should be used when you want a default value in scenarios where the original value is null or undefined. |
Logical OR Operator (||) | This operator is more appropriate under circumstances where you wish to have a fallback value not just for null or undefined, but also for ”, 0, NaN, and false. |
Let’s delve into a more comprehensive understanding on these two JavaScript operators that are frequently used for providing an alternative or fallback value.
The Nullish Coalescing Operator (??) is a recent addition to JavaScript, introduced as part of ES2020 revision. This operator is highly applicable when your code requires an alternative whenever the original value is either null or undefined. The symbol “??” represents this operator.
This allows it to handle absent variables gracefully by returning a given default object or value. The application of the nullish coalescing operator can be elucidated with a piece of example code:
let name = user.name ?? 'Guest'; // If user.name is null or undefined, 'Guest' is returned
Contrarily, the JavaScript Logical OR Operator (||), apart from null and undefined, also considers empty string (”), NaN, zero, and boolean false as falsy values and provides the fallback if any of these values appear. It translates into ‘provide an alternative if the initial value isn’t truthy’. Here’s how || works in practice:
let name = user.name || 'Guest'; // If user.name is null, undefined, empty string, 0, NaN or false, 'Guest' is returned
The one important thing to note is that while nullish coalescing operator only contemplates explicit “nothingness”, identified by null and undefined, the logical OR considers more values as “falsey”. Hence, the choice of these operators should be contextual, based on an understanding of what qualifies as ‘unwanted’ or ’empty’ within your application context.
As famously said by Angus Croll, a well-known JavaScript Developer, “Choose your defaults wisely, they reflect the heart of your coding style.”
Choosing between the Logical OR Operator and Nullish Coalescing Operator ultimately depends on how you want to handle the falsy values in your code. If you wish to provide fallbacks for all non-truthy types, || would work best. However, if fallbacks are needed only for null or undefined, ?? turns out to be your go-to operator.
Understanding the Basics of Nullish Coalescing and Logical OR
The `Nullish Coalescing` operator (`??`) and `Logical OR` operator (`||`) are both used to provide default values in JavaScript. However, they behave differently when it comes to certain “falsy” values, and understanding these differences is crucial when determining which operator to use.
To help us explore their behaviour, let’s consider the following scenario:
html
let value = variable ?? 'default'; let value2 = variable2 || 'default';
Here are some key points about the Nullish Coalescing operator:
– The `Nullish Coalescing` operator (`??`) checks if a value is null or undefined.
– If the value is either null or undefined, it will return the value after the `??`, otherwise it returns the original value.
– It treats zero (`0`), empty string (`””`), and `false` as legitimate values, hence does not default for these.
In respect to the Logical OR operator:
– The `Logical OR` operator (`||`) checks if a value is falsy.
– If the value is falsy (which includes false, 0, “”, null, undefined, and NaN), it will return the value after the `||`. Otherwise, it will return the original value.
– So, unlike `??`, it doesn’t consider `0`, `””`, `false` as legitimate, defaulting even on these values.
To answer your question specifically on when one should be preferred over the other, consider the nature of the values you’re dealing with:
Scenario | Choice |
---|---|
If the code needs to regard `0`, `false`, and `””` as legitimate values | Use `Nullish Coalescing` (`??`) |
If the code needs to default for `0`, `false`, and `””` beside null or undefined | Use `Logical OR` (`||`) |
Why does this matter? Providing inappropriate default values can lead to bugs which are hard to trace, making one’s code unreliable or even unpredictable.
As Kyle Simpson, noted developer and author of the You Don’t Know JS book series, puts it, “No matter how much experience you have or think you have, if you’re not careful and disciplined, forgetting a single `null` check… can bring down your whole program”(source). In line with this, knowing when to use `??` over `||` (and vice versa) becomes highly essential in maintaining resilient code.
Deciphering Use Cases for (Nullish Coalescing) in JavaScript
The Nullish Coalescing operator (??) and the Logical OR (||) operator in JavaScript have their special uses in coding scenarios. Understanding when to use one over the other is key to writing efficient code.
The methodologies employed by them in analyzing variables are quite different:
Operator | Methodology |
---|---|
Nullish Coalescing (??) | Returns the right operand if the left is null or undefined |
Logical OR (||) | Returns the right operand if the left is falsy (i.e., false, 0, “”, null, undefined, NaN) |
Let’s breakdown specific scenarios where each would be used:
Use Cases for Nullish Coalescing
let value = input ?? "default";
In this case, the Nullish Coalescing operator checks whether the ‘input’ variable is either null or undefined. If it is, it then assigns the string “default” to the ‘value’ variable. This operator ignores other “falsy” values like 0 or an empty string.
This can be especially useful in situations where you might have integer values that could be zero, and a fallback default value should only be used if the initial value is null or undefined.
Use Cases for Logical OR
let status = message || "No new messages";
The Logical OR operator, on the other hand, considers all “falsy” values. Beyond null and undefined, it includes false, 0, “”, and NaN. If the ‘message’ variable contains any of these values, ‘status’ will be assigned the value “No new messages”.
This particular use case is handy when you want to provide a default value for empty strings or other “falsy” values.
As Douglas Crockford once said, “JavaScript is the world’s most misunderstood programming language”. Learning how and when to use these operators effectively can help unravel some of this misunderstanding, leading to more efficient and understandable code. Source
Exploring Advantageous Scenarios for Using || (Logical Or)
When contemplating the advantageous scenarios for using
|| (Logical Or)
, it can be beneficial to evaluate these in conjunction with the usage of
?? (Nullish Coalescing)
. Both serve as efficient techniques for providing default values, yet their applications vary based on distinct operational parameters.
Operator | Description |
---|---|
|| (Logical OR) |
Returns the first truthy value or the last value if all are falsy. Treats ‘0’, ”, null, undefined, NaN, and false as falsy. |
?? (Nullish Coalescing) |
Returns the first value that isn’t null or undefined. Allows ‘0’, ” (empty string), NaN, and false as valid values. |
The
LOR
operator is quite salient when there’s a need to return the first “truthy” value from a list or default to a certain value when the variables are “falsy”. For instance:
var setting = userSetting || defaultSetting;
In the case that “userSetting” holds a “falsy” value such as false, null, or undefined, our code will resort to the “defaultSetting”, thus averting potential errors.
Contrarily, when you desire to accept all values, including the JavaScript “falsy” values except for null or undefined, the
Nullish Coalescing
operator is the superior choice. Code snippet illustration:
var voteCount = userVotes ?? minimumVotes;
In this particular example, even if “userVotes” holds a value of ‘0’ (which is “falsy”), it would still be considered and returned by the code, which stems from the distinct method of operation of the Nullish Coalescing operator.
From MDN Web Docs, one can find that ‘nullish values are those which evaluate to null or undefined’, contrasting to ‘falsy values’ construed in the broader JavaScript context. This affirms that adapting the approach towards using either
||
, or
??
is ultimately determined by both your data structure and individual goals.
As Brenna O’Brien quotes, “Coding isn’t about typing lines of code. It’s about expressing ideas.” As such, while choosing between
|| (Logical Or)
and
?? (Nullish Coalescing)
, it is about how you want to articulate your logic in your code.
Key Differences Between Nullish Coalescing Vs Logical OR
The choice between Nullish Coalescing and Logical OR in JavaScript often depends on the precise requirements of the situation. Both operators are used as default value provision strategies when working with possibly undefined or null values, but they behave differently due to how they operate on “falsy” values.
Nullish Coalescing (??) | Logical OR (||) | |
---|---|---|
Falsy Values handling | Only reacts to undefined and null | Reacts to all javascript falsey values |
Appropriate Usage | When you want to consider only null or undefined as “default” trigger points. | When dealing with possible falsy JavaScript values like an empty string “”, number 0, NaN, and false. |
Now considering these specifications for the correct situations, let’s focus on ‘when’ to use each of them:
Use Nullish Coalescing – ??
If you want to provide a default value for a variable and care specifically about null or undefined, then nullish coalescing is the correct choice. It allows you to keep zero, an empty string (“”) or other JavaScript symbols in your code that may be considered falsy with || operator, but actually carry meaning in your program.
let score = 0; let displayedScore = score ?? 100; // Score: 0, because '0' isn't null or undefined.
Use Logical OR – ||
But, if you need a default value for a variable that might hold any falsy JavaScript values like an empty string “” or the number 0, then logical OR can prove more beneficial.
let name = ""; let greeting = name || "Guest"; // Greeting: 'Guest', because "" is a falsy value in JavaScript.
As Tom Catchesides rightly said, “Language features don’t exist in isolation: they should be fit into the language as a whole by interacting with other features.” So choosing Nullish Coalescing vs Logical OR should not just be about ‘what’ they do, but correctly judging ‘when’ and ‘where’ to employ them based on their specific attributes and your requirement for handling “default” scenarios. This analysis empowers developers to make reasonable and preferable syntax decisions along the way. Here’s more on the topic from an informative article from [MDN Docs].
The choice between using Nullish Coalescing (`??`) and Logical Or (`||`) in JavaScript is one that hinges on the specific needs of your application and how it handles “falsy” values.
Table for comparison:
Nullish Coalescing (`??`) | Logical Or (`||`) | |
---|---|---|
Falsy Values | Ignores falsy values except `null` and `undefined` | Treats all falsy values as equivalent to `false` |
Use case | When you need a default value only for `null` or `undefined` | When you desire a default value for any falsy value including `””`, `0`, `NaN`, `false`, `null`, `undefined` |
With the
??
operator, the expression will return the right-hand side operand if the left-hand side operand is `null` or `undefined`. Other “falsy” values such as `false`, `0`, `NaN`, empty string(`””`) will not trigger a fallback to the default value.
On the other hand, the
||
operator considers any ‘falsy’ value (such as `false`, `0`, `””`, `null`, `undefined`, and `NaN`) as triggering condition to fall back to the default value.
Mozilla’s JavaScript Reference is an insightful guide on understanding how these two operators function.
An interesting perspective is this quote by the renowned developer, Douglas Crockford, “JavaScript’s loose type system allows us to use `null` and `undefined` interchangeably, but operational correctness becomes paramount in writing robust code. Be judicious when choosing between Nullish Coalescing and Logical OR.”
In brief, the choice between Nullish Coalescing (`??`) and Logical Or (`||`) largely rest on the specific requirements around falsy value handling within your JavaScript application. It underlines the importance of understanding the nuances of these two operators for writing more precise and bug-free code.