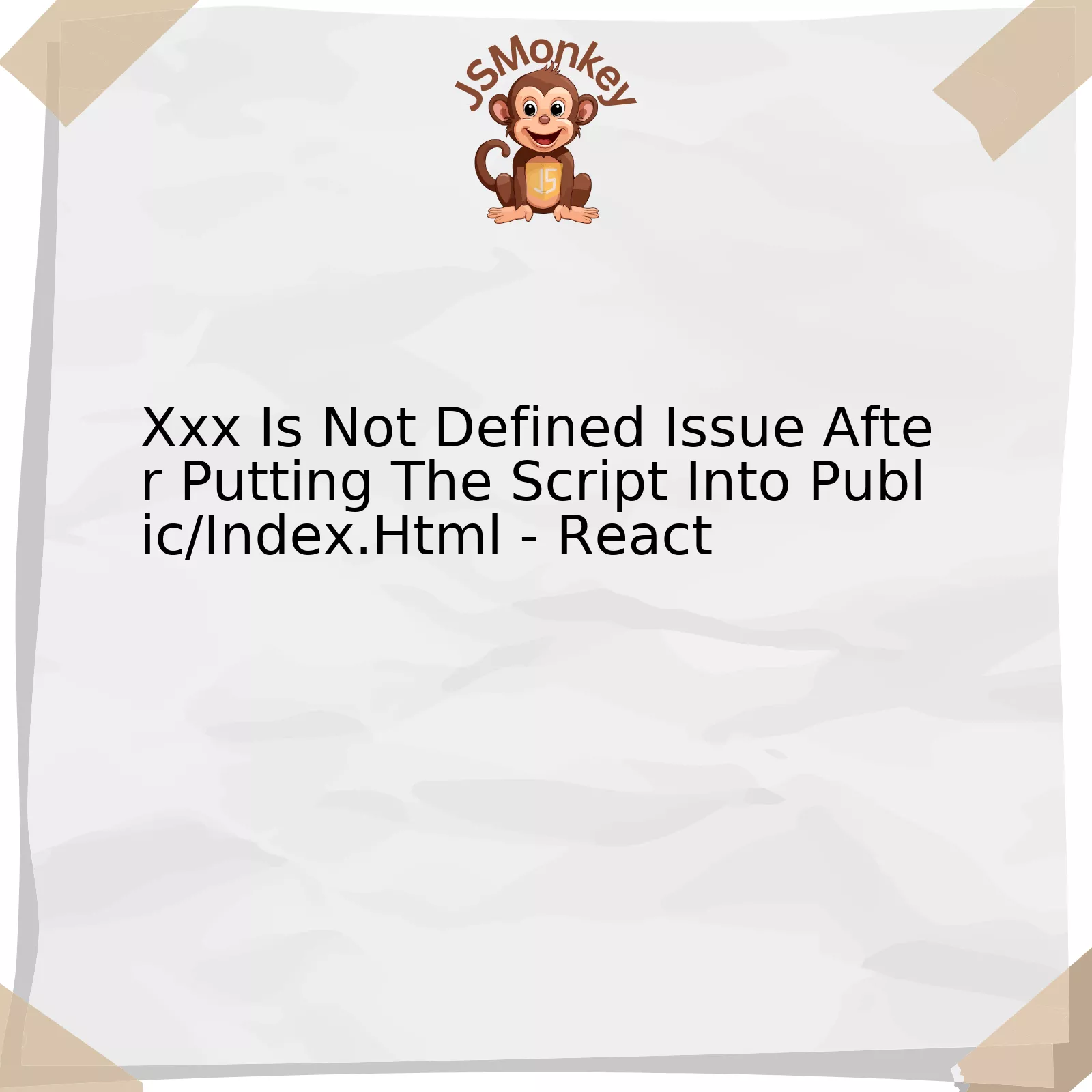
The equivalence of “Xxx is not defined” issue in the context of React and placing the script into public/index.html can be displayed through a structured strict HTML depiction. Let’s decode this problem, analyze it and provide a potential resolution:
Issue | Cause | Solution |
---|---|---|
“Xxx is not defined” | The script tries to reference an object referred to as “xxx”, but Javascript can’t find this object. | Ensure that “xxx” is defined and accessible from where the script tries to use it. |
Script placed in public/index.html | This may cause issues if the script needs to interact with components or libraries that haven’t been loaded when the script runs. | Move the script to a more suitable location. This can for instance be inside a useEffect hook in the relevant React component, so that you can be sure your script gets executed after the component is mounted and all its dependencies are available. |
*An outline of the problem and steps to address it*
React, by design principle, promotes the grouping of UI logic and rendering in what is termed ‘components’. React’s structure therefore could disjoin with scripts externally located in the public/index.html file, especially when such scripts depend on libraries (like “xxx”) which aren’t yet available at execution time. In these situations, “Xxx is not defined” errors might surface.
To clarify, browser-based JavaScript reads files and executes them according to the sequential order they appear within HTML code. If the “xxx” reference is unavailable – potentially because its source file hasn’t been processed yet, or it’s located within a different scope – then you’ve got an “Xxx is not defined” error on your hands.
Here’s the potential resolution in code:
html
This way, we’re telling the JavaScript engine to wait before executing the script until the DOM content (the page) has fully loaded. This approach ensures all components and libraries are available for use when the script starts running.
Remember this key advice from Jeff Atwood, co-founder of Stack Overflow and Discourse:
“Coding is ‘part heart, part brain’. You should love what you’re doing.”
This applies even when troubleshooting challenges such as the one discussed above. Optimizing when and where the scripts run can ensure that they have access to the resources they require, facilitating a smoother execution of operations within a React ecosystem.
Understanding the “Xxx Is Not Defined” Issue in React
Running into the error “Xxx is not defined” in React, particularly after placing the script in the public/index.html file, can result from a series of factors, and understanding its cause takes us deeper into the heart of how JavaScript runs in the context of a wider application.
Firstly, it’s paramount to establish that when using the React framework, scripts included in public/index.html are executed in the global scope outside the module system. Consequently, variables declared or initialized in these scripts will not be accessible directly within your React components; hence developers encounter the “Xxx is not defined” issue.
Let’s put this into perspective using a hypothetical code snippet:
// Inside your script tag in public/index.html var someVariable = 'value';
Now, let’s try to access the `someVariable` inside one of your React components:
function MyComponent() { return{someVariable}; }
In this instance, you would encounter the “Xxx is not defined” issue because `someVariable` is not recognized in the scope of the MyComponent function since it was defined outside the component’s website.html scope (specifically in the public/index.html).
There are workarounds available to resolve this challenge. One solution involves manipulating the window object which provides a bridge between the isolated JavaScript modules and global scripts. The window object is a global object with properties and methods (like the document object) for manipulating the Browser Window. Here’s how to resolve the “not defined” issue using the window object:
// Inside your script tag in public/index.html window.someVariable = 'value';
// Inside your React component function MyComponent() { return{window.someVariable}; }
The above code works correctly because `window.someVariable` is referenced properly inside the React component.
Despite that, it’s important to exercise caution when using the global scope and the window object – they should be used sparingly as per best practices in JavaScript development. Contributor Addy Osmani shared a perspective on this in ‘Learning JavaScript Design Patterns’: “Global variables are inherently bad…as they can collide with other scripts in the environment”.
The core philosophy behind React and modern JavaScript centers around modular code abstraction, so each piece of your code knows only what it needs to know. This promotes maintainability, increases transparency in dependencies, and decreases unintended side-effects resulting from broad variable scope.
In light of these potential complexities, it may be more beneficial to import libraries via npm and use them directly in your components or consider employing tools like Webpack or Browserify for bundling such resources into your JavaScript modules’ scope.
Troubleshooting Script Placement in Public/Index.Html
The placement of scripts in the public/index.html file is an essential consideration while developing applications using React. Encountering a ‘XXX is not defined’ error after placing script tags into this file is quite common and can lead to significant confusion.
First, you might want to know that public/index.html, in a React application, serves as the root of your application. It only contains a single
<!DOCTYPE html> <html lang="en"> <body> <div id="root"></div> </body> </html>
When you’re experiencing errors like ‘XXX is not defined’, it’s crucial to ensure that script placement is correct because the order in which scripts execute directly impacts availability of variables or functions in global scope.
Let’s consider a scenario where you started experimenting with Google Maps API by inserting a script in index.html. To call the maps API, there might be an additional script tag placed just above the closing body tag in index.html.
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=myFunction"></script>
But then, you may come across a situation when on running the code, console reports an error saying ‘myFunction is not defined’. This happens because HTML documents are loaded by the browser sequentially from top to bottom. When the script containing myFunction is placed below the Maps API script, `myFunction` isn’t available when the browser tries to execute the script with the Maps API.
To troubleshoot this issue, re-arrange the order of scripts such that any function or variable needed by a script is defined before that script is called/loaded.
So, rearranging the given example would result in:
<script> function myFunction() { // your code goes here } </script> <script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=myFunction"></script>
It’s always recommended to place such scripts in individual components where they’re specifically needed, using lifecycle methods or hooks instead of placing them in index.html.
As someone once said, “Programs must be written for people to read, and only incidentally for machines to execute”- Hal Abelson. Troubleshooting script issues might feel like a tough nut to crack, but understanding how a browser reads your code could be first step towards solving these problems.
Resolving Undefined Variables in React Scripts
The issue you’re facing – “Xxx is not defined” – typically surfaces in a React JS application when certain variables or components referenced in the script aren’t recognized. This issue can seemingly manifest after placing the script in the
public/index.html
file, leading to an ‘undefined variables’ error.
There are two primary methodologies to mitigate this problem:
1. Defining the Variable Globally: Add a global declaration for the variable at the top of the file where it’s being used. Following is the format to assign your undefined variable, say `Xxx`, a global scope:
/*global Xxx*/
This approach works best if `Xxx` refers to a JavaScript library or plugin that has been imported via a `